PDF Signatures#
Signatures#
There are two distinct signature types which can be used with App Kit.
Digital signatures can be placed as signing areas in documents and also signed, whereas E-signatures can just be placed onto a document as part of a signing activity. E-signatures are the simplest use case and just involve a hand drawn signature without digital ID certification.
Digital Signatures#
Placing a Digital Signature#
Just like regular annotations, the annotating mode within the document’s view controller needs to be set.
To turn on digital signature mode set the annotating mode to MuPDFDKAnnotatingMode_DigitalSignature
within your MuPDFDKBasicDocumentViewController
instance. To exit this mode then set the annotating mode to MuPDFDKAnnotatingMode_None
.
When in digital signature mode if the user taps the document then a digital signature area will be placed at that point, it can be resized and moved to the desired position, but once the document is saved then the position is set and the digital signature field becomes uneditable.
basicDocVc.annotatingMode = MuPDFDKAnnotatingMode_DigitalSignature
basicDocVc.annotatingMode = MuPDFDKAnnotatingMode_DigitalSignature;
Signing a Digital Signature#
Digital signatures use certificate-based digital IDs to authenticate signer identity and demonstrate proof of signing by binding each signature to the document with encryption.
If there is a set digital signature area on your document then tapping that will invoke App Kit to display a pre-built UI for the signing activity. This is a carefully considered UI and will not allow you to continue until you have imported a valid digital ID.
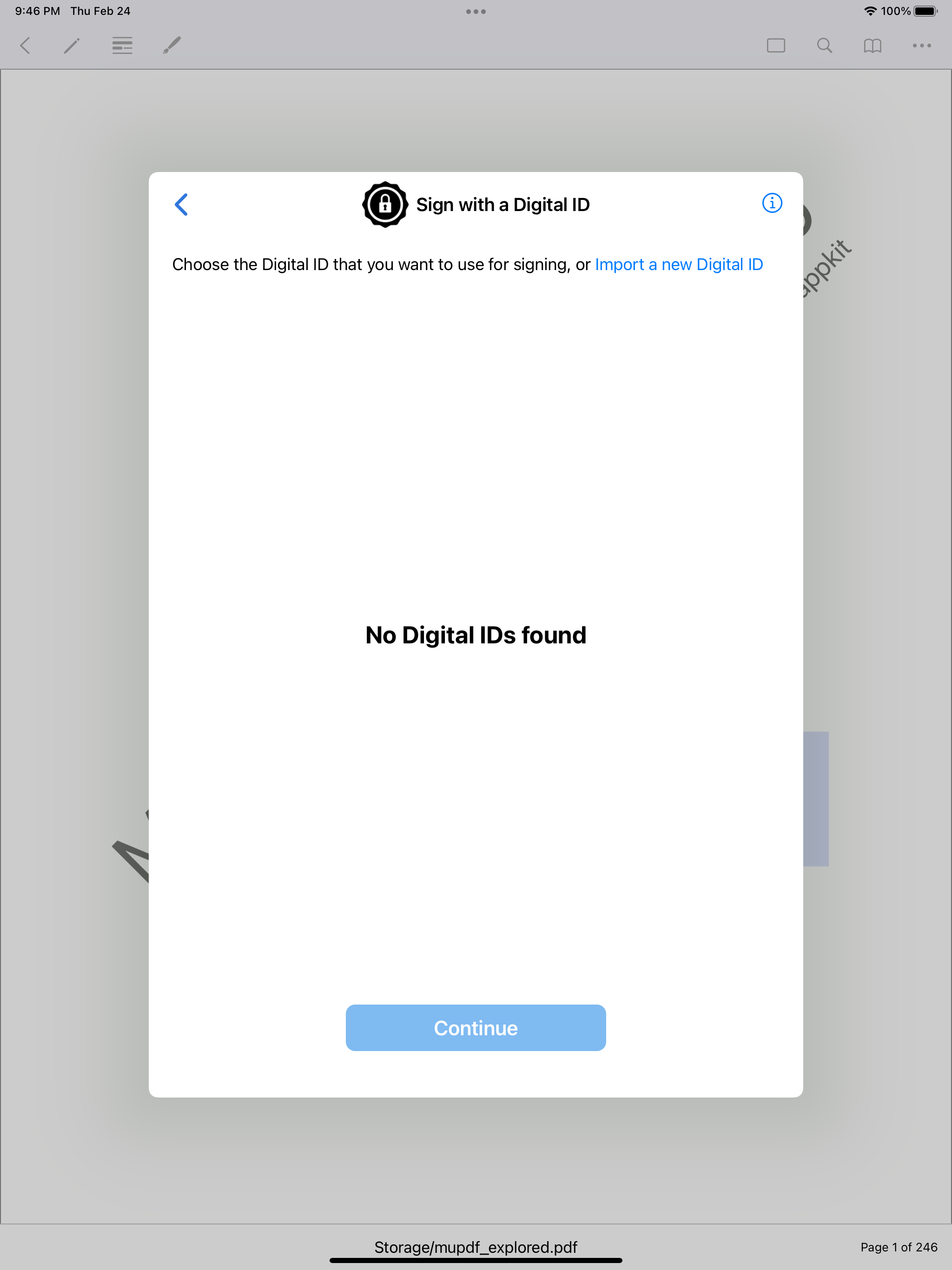
The UI for digital signing
E-signatures#
E-signatures should have been created (i.e. drawn by the user) before they are placed and there is some handy drop-in UI built into App Kit which allows for this. On your document view controller instance you can invoke the following segue to make a dialog appear:
self.performSegue(withIdentifier: "esignature-place-edit", sender: nil)
[self performSegueWithIdentifier:@"esignature-place-edit" sender:nil];
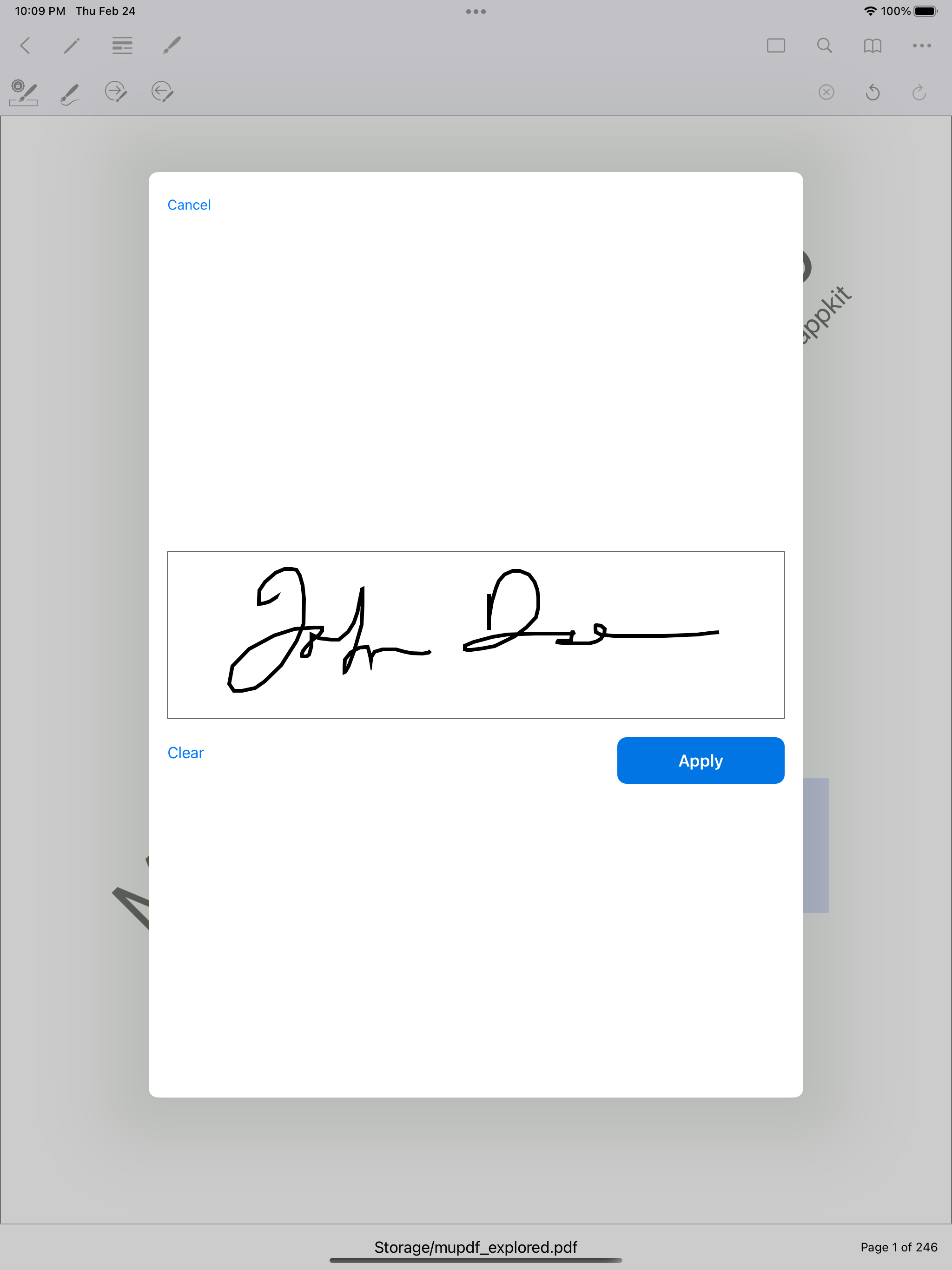
The UI for creating your e-signature
The E-signatures Drop-In UI#
To pick up key events from the e-signatures drop-in UI, your document view controller code should listen to the unwind seques from the e-signature storyboard and act accordingly.
@IBAction func unwindForEditESignature(_ unwindSegue:UIStoryboardSegue) {
/// User asked to edit(draw) the e-signature
let segue = unwindSegue as! ARDKSegueWithCompletion
segue.completion = {[weak self] in
self?.performSegue(withIdentifier:"signature-draw", sender: nil)
}
}
@IBAction func unwindForPlaceESignature(_ unwindSegue:UIStoryboardSegue) {
/// User asked to place an e-signature
if documentViewController.eSigImage != nil {
documentViewController.annotatingMode = MuPDFDKAnnotatingMode_ESignature
}
}
@IBAction func unwindForCancel(_ unwindSegue:UIStoryboardSegue) {
/// User chose cancel
}
@IBAction func signatureDrawnUnwindAction(_ unwindSegue:UIStoryboardSegue) {
/// set the e-signature image on the document view controller
let vc = unwindSegue.source as! ARDKSignaturesDrawViewController
documentViewController.eSigImage = vc.image
}
@IBAction func signatureDrawCancelAction(_ unwindSegue:UIStoryboardSegue) {
}
- (IBAction)unwindForEditESignature:(UIStoryboardSegue *)unwindSegue
{
ARDKSegueWithCompletion *segue = (ARDKSegueWithCompletion *)unwindSegue;
__weak typeof(self) weakSelf = self;
segue.completion = ^{
[weakSelf performSegueWithIdentifier:@"signature-draw" sender:nil];
};
}
- (IBAction)unwindForPlaceESignature:(UIStoryboardSegue *)unwindSegue
{
if (documentViewController.eSigImage != nil) {
documentViewController.annotatingMode = MuPDFDKAnnotatingMode_ESignature;
}
}
- (IBAction)unwindForCancel:(UIStoryboardSegue *)unwindSegue
{
}
- (IBAction)signatureDrawnUnwindAction:(UIStoryboardSegue *)unwindSegue
{
ARDKSignaturesDrawViewController *vc = (ARDKSignaturesDrawViewController *)unwindSegue.sourceViewController;
documentViewController.eSigImage = vc.image;
}
-(IBAction)signatureDrawCancelAction:(UIStoryboardSegue*)unwindSegue
{
}
Setting an E-signature Image#
To set the image used for an e-signature then access the eSigImage
property within your MuPDFDKBasicDocumentViewController
instance to a valid UIImage
.
documentViewController.eSigImage = UIImage
documentViewController.eSigImage = UIImage;
Placing an E-signature#
To turn on e-signature mode set the annotating mode to MuPDFDKAnnotatingMode_ESignature
within your MuPDFDKBasicDocumentViewController
instance. To exit this mode then set the annotating mode to MuPDFDKAnnotatingMode_None
.
When in e-signature mode if the user taps the document then an e-signature area will be placed at that point, it can be resized and moved to the desired position. Unlike digital signatures, an e-signature will always be able to be edited even after a document has been saved.
basicDocVc.annotatingMode = MuPDFDKAnnotatingMode_ESignature
basicDocVc.annotatingMode = MuPDFDKAnnotatingMode_ESignature;
Querying Signature Modes#
To query for signature mode an application developer should simply query the annotation mode of the document view controller. If the mode returns MuPDFDKAnnotatingMode_DigitalSignature
or MuPDFDKAnnotatingMode_ESignature
then there is currently a signature mode in progress.
Searching for signatures#
If you have a document with multiple digital signatures you can query the document to search through and focus those signatures.
Searching for signatures involves invoking the findSigStart
method with a designated callback which is responsible for keeping track of the page number and annotation identifier as results come through from the document. Thus, specific class member variables which keep track of these variables must be designated before the signature searching starts. Once a signature is found the callback mechanism should be responsible for focusing the outline of the signature as well as moving the document view controller to the location of the signature.
To start the search use the following code with MuPDFDKSearchDirection
being set to either MuPDFDKSearch_Forwards
or MuPDFDKSearch_Backwards
:
var sigSearchPage:Int = -1
var sigSearchAnnot:Int = -1
...
func searchForSignature(direction:MuPDFDKSearchDirection) {
var cancelled:ObjCBool = false
doc.findSigStart(atPage: sigSearchPage,
andAnnot: sigSearchAnnot,
in: direction,
cancelFlag: &cancelled,
onEvent: { event, page, annot, rect in
switch event {
case MuPDFDKSearch_Found:
self.sigSearchPage = page
self.sigSearchAnnot = annot
self.documentViewController.session.doc.outlineArea(rect, onPage: page)
self.documentViewController.showArea(rect, onPage: page)
case MuPDFDKSearch_Progress:
case MuPDFDKSearch_Cancelled:
default:
()
}
})
}
NSInteger sigSearchPage = -1;
NSInteger sigSearchAnnot = -1;
...
- (void)searchForSignature:(MuPDFDKSearchDirection)direction
{
BOOL cancelled;
[doc findSigStartAtPage:sigSearchPage
andAnnot:sigSearchAnnot
inDirection:direction
cancelFlag:&cancelled
onEvent:^(MuPDFDKSearchEvent event, NSInteger page, NSInteger annot, CGRect rect) {
switch (event)
{
case MuPDFDKSearch_Found:
self.sigSearchPage = page;
self.sigSearchAnnot = annot;
[self.documentViewController.session.doc outlineArea:rect onPage:page];
[self.documentViewController showArea:rect onPage:page];
case MuPDFDKSearch_Progress:
case MuPDFDKSearch_Cancelled:
default:
break;
}
}
];
}
Note
Searching for signatures only looks for digital signature fields. If there are e-signatures placed on the document these will be ignored.
This software is provided AS-IS with no warranty, either express or implied. This software is distributed under license and may not be copied, modified or distributed except as expressly authorized under the terms of that license. Refer to licensing information at artifex.com or contact Artifex Software, Inc., 39 Mesa Street, Suite 108A, San Francisco, CA 94129, USA, for further information.